Monitoring a Humid Basement with the DHT22, RaspberryPi, and Flask
Humidity and basements go hand in hand. Monitoring levels can tell you if you should be seeking a remedy like a dehumidifier.
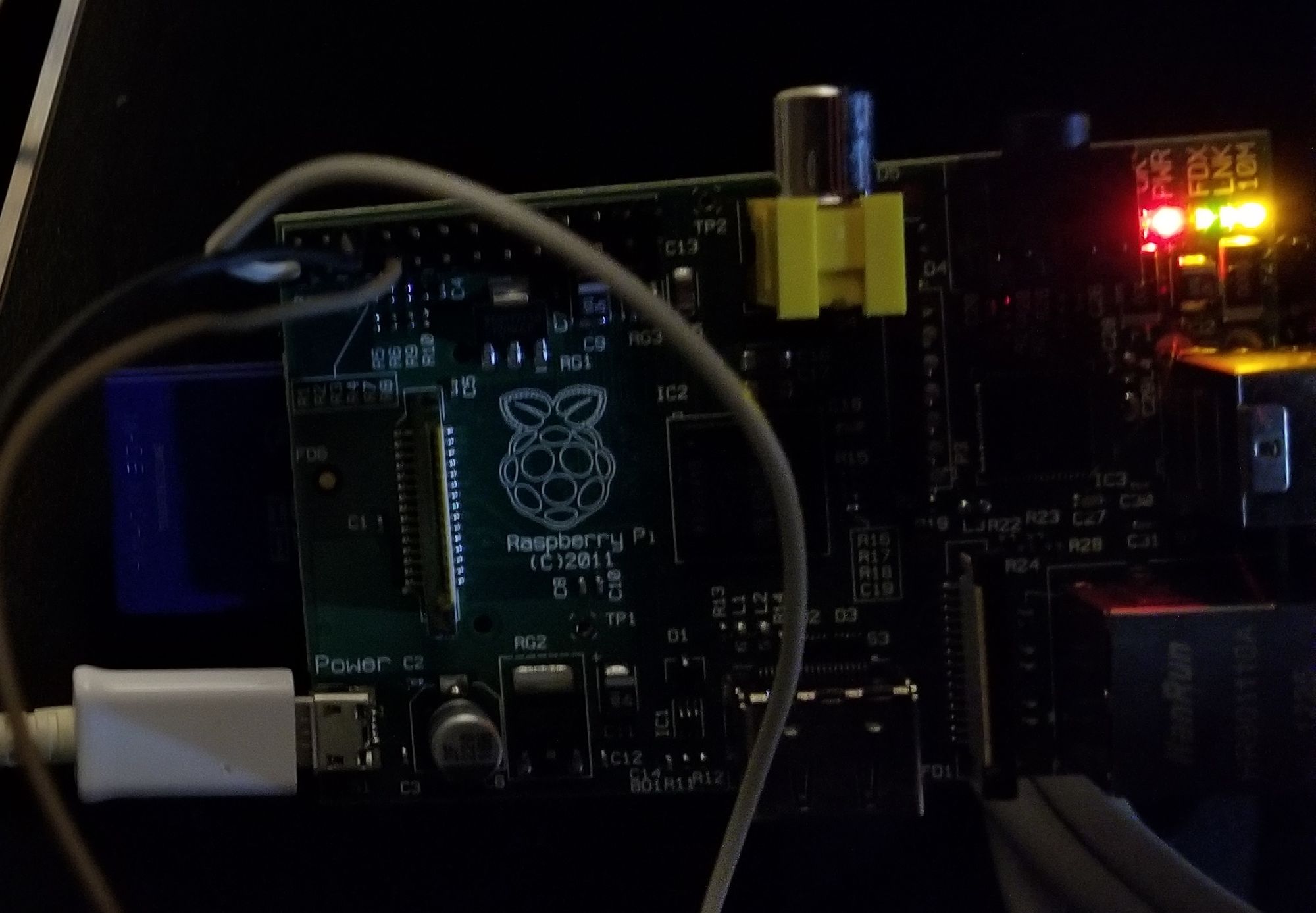
Git repository for this post: https://github.com/jaredmoore/hyperion/
A humid basement can be a nightmare to a new homeowner. When touring our new home we immediately noticed a musty smell in the basement and the air was quite heavy. Being a bit of a data nut and a programmer, I knew I would have to start monitoring the temperature and humidity to assess the situation. After looking into the various options available to me, I decided to embark on a journey of creating my own custom home monitoring solution.
The first step was to investigate different options for monitoring temperature and humidity. I had an old RaspberryPi from 2011 laying around to act as the base for the monitoring. My goals for this initial system were:
- Periodically sample temperature and humidity.
- Record the data in a flat file.
- Serve the data locally and plot it with D3.js or similar library.
Periodically Sample Temperature and Humidity
Searching on Amazon, I found a pair of DHT22 Temperature Humidity Sensors. Although there were only a few reviews, it seemed that they were preferable to the DHT11 sensors. I'm not terribly concerned with accuracy as a ballpark range is fine for the application. Hooking the sensor up to the RaspberryPi was straightforward after following the tutorial found at "Raspberry Pi: Measure Humidity and Temperature with DHT11/DHT22".
On the software side, I used the Adafruit Python DHT Library and built a custom script to poll the sensor every ten minutes. Setup for the code can be found in lines 27 to 43 of monitor.py
. Code for the polling is below:
print('Logging sensor measurements to {0} every {1} seconds.'.format(LOGFILE, FREQUENCY_SECONDS))
print('Press Ctrl-C to quit.')
while True:
# Attempt to get sensor reading.
humidity, temp = Adafruit_DHT.read(DHT_TYPE, DHT_PIN)
# Skip to the next reading if a valid measurement couldn't be taken.
# This might happen if the CPU is under a lot of load and the sensor
# can't be reliably read (timing is critical to read the sensor).
if humidity is None or temp is None:
time.sleep(2)
continue
print(datetime.datetime.now(tz).strftime('%Y-%m-%d %H:%M:%S'))
print('Temperature: {0:0.1f} C'.format(temp))
print('Humidity: {0:0.1f} %'.format(humidity))
# Append the data in the spreadsheet, including a timestamp
with open(LOGFILE,'a') as f:
f.write(datetime.datetime.now(tz).strftime('%Y-%m-%d %H:%M:%S')+',')
f.write(str(temp)+',')
f.write(str(humidity)+"\n")
# Wait 30 seconds before continuing
print('Wrote a row to {0}'.format(LOGFILE))
time.sleep(FREQUENCY_SECONDS)
Currently, the code writes to a local log file creating data for time, temperature and humidity. The script is launched and allowed to run in the background. In the future, I plan to add this to an automated launching script so that it starts upon rebooting the Pi.
Serve the data locally and plot it with D3.js
My next goal was to create a simple local webserver in order to plot the data collected by the Pi. Following the d3-flask-blog-post repository, I went about creating my flask application.
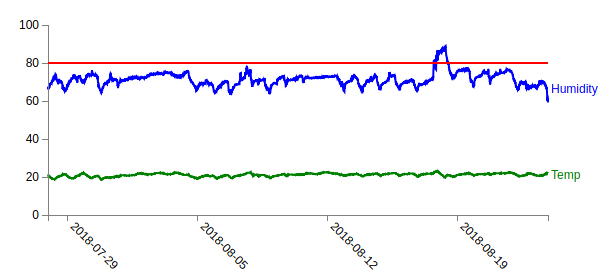
My flask server contains a single index.html
page. It serves a line plot showing the humidity, temperature, and red line at 80% humidity. Styling will be updated in the future.
For now I have assigned the RaspberryPi a static IP on my local network and I can access it through any of my devices.
Conclusion
So far this has been a fairly inexpensive project but it is generating the data I am interested in seeing. Aside from a few very hot days where we also opened the windows, humidity in the basement is staying under the critical zone, although still higher than I would like. After seeing this data, a dehumidifier will be purchased in the near future.